4. Spring MVC form validation
Till now our example application is able to display form, and receiving submitted form with filled values. In real life application, user’s will make lots of mistake in filling forms. Validation should always be on client side, but to protect data integrity you should also validate data on server side as well.
Validation can be added into application into two steps i.e. first at view layer and then at controller code.
4.1. Modified addEmployee.jsp
<%@ page contentType= "text/html;charset=UTF-8" %> <%@ taglib prefix= "form" uri= "http://www.springframework.org/tags/form" %> <%@ taglib prefix= "spring" uri= "http://www.springframework.org/tags" %> <html> <head> <title>Add Employee Form</title> <style> .error { color: #ff0000; font-weight: bold; } </style> </head> <body> <h2><spring:message code= "lbl.page" text= "Add New Employee" /></h2> <br/> <form:form method= "post" modelAttribute= "employee" > <%-- <form:errors path= "*" cssClass= "error" /> --%> <table> <tr> <td><spring:message code= "lbl.firstName" text= "First Name" /></td> <td><form:input path= "firstName" /></td> <td><form:errors path= "firstName" cssClass= "error" /></td> </tr> <tr> <td><spring:message code= "lbl.lastName" text= "Last Name" /></td> <td><form:input path= "lastName" /></td> <td><form:errors path= "lastName" cssClass= "error" /></td> </tr> <tr> <td><spring:message code= "lbl.email" text= "Email Id" /></td> <td><form:input path= "email" /></td> <td><form:errors path= "email" cssClass= "error" /></td> </tr> <tr> <td colspan= "3" ><input type= "submit" value= "Add Employee" /></td> </tr> </table> </form:form> </body> </html> |
You will also need to update message resource file.
lbl.page=Add New Employee lbl.firstName=First Name lbl.lastName=Last Name lbl.email=Email Id //Error messages error.firstName=First Name can not be blank error.lastName=Last Name can not be blank error.email=Email Id can not be blank |
4.2. Modified submitForm() method
@RequestMapping (method = RequestMethod.POST) public String submitForm( @ModelAttribute ( "employee" ) EmployeeVO employeeVO, BindingResult result, SessionStatus status) { //Validation code start boolean error = false ; System.out.println(employeeVO); //Verifying if information is same as input by user if (employeeVO.getFirstName().isEmpty()){ result.rejectValue( "firstName" , "error.firstName" ); error = true ; } if (employeeVO.getLastName().isEmpty()){ result.rejectValue( "lastName" , "error.lastName" ); error = true ; } if (employeeVO.getEmail().isEmpty()){ result.rejectValue( "email" , "error.email" ); error = true ; } if (error) { return "addEmployee" ; } //validation code ends //Store the employee information in database //manager.createNewRecord(employeeVO); //Mark Session Complete status.setComplete(); return "redirect:addNew/success" ; } |
5. Spring MVC example – Demo
Before testing let’s add other essential files.
5.1. Spring mvc configuration file
< beans xmlns = "http://www.springframework.org/schema/beans" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xmlns:context = "http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context/ http://www.springframework.org/schema/context/spring-context-3.0.xsd"> < context:component-scan base-package = "com.howtodoinjava.demo" /> < bean class = "org.springframework.web.servlet.mvc.annotation.AnnotationMethodHandlerAdapter" /> < bean class = "org.springframework.web.servlet.mvc.annotation.DefaultAnnotationHandlerMapping" /> < bean class = "org.springframework.web.servlet.view.InternalResourceViewResolver" > < property name = "prefix" value = "/WEB-INF/views/" /> < property name = "suffix" value = ".jsp" /> </ bean > < bean id = "messageSource" class = "org.springframework.context.support.ResourceBundleMessageSource" > < property name = "basename" value = "messages" /> </ bean > </ beans > |
5.2. web.xml
< web-app id = "WebApp_ID" version = "2.4" xmlns = "http://java.sun.com/xml/ns/j2ee" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd"> < display-name >Spring Web MVC Hello World Application</ display-name > < servlet > < servlet-name >spring</ servlet-name > < servlet-class > org.springframework.web.servlet.DispatcherServlet </ servlet-class > < load-on-startup >1</ load-on-startup > </ servlet > < servlet-mapping > < servlet-name >spring</ servlet-name > < url-pattern >/</ url-pattern > </ servlet-mapping > </ web-app > |
5.3. pom.xml
< project xmlns = "http://maven.apache.org/POM/4.0.0" xmlns:xsi = "http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation = "http://maven.apache.org/POM/4.0.0 http://maven.apache.org/maven-v4_0_0.xsd" > < modelVersion >4.0.0</ modelVersion > < groupId >com.howtodoinjava.demo</ groupId > < artifactId >springmvcexample</ artifactId > < packaging >war</ packaging > < version >1.0-SNAPSHOT</ version > < name >springmvcexample Maven Webapp</ name > < url >http://maven.apache.org</ url > < dependencies > < dependency > < groupId >junit</ groupId > < artifactId >junit</ artifactId > < version >4.12</ version > < scope >test</ scope > </ dependency > <!-- Spring MVC support --> < dependency > < groupId >org.springframework</ groupId > < artifactId >spring-webmvc</ artifactId > < version >4.1.4.RELEASE</ version > </ dependency > < dependency > < groupId >org.springframework</ groupId > < artifactId >spring-web</ artifactId > < version >4.1.4.RELEASE</ version > </ dependency > <!-- Tag libs support for view layer --> < dependency > < groupId >javax.servlet</ groupId > < artifactId >jstl</ artifactId > < version >1.2</ version > < scope >runtime</ scope > </ dependency > < dependency > < groupId >taglibs</ groupId > < artifactId >standard</ artifactId > < version >1.1.2</ version > < scope >runtime</ scope > </ dependency > </ dependencies > < build > < finalName >springmvcexample</ finalName > </ build > </ project > |
Now test the application.
1) Enter URL : http://localhost:8080/springmvcexample/employee-module/addNew : It will display blank form.
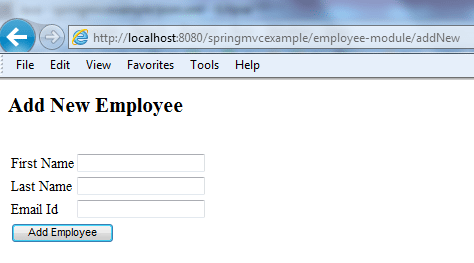
2) Fill first name field and hit “Add Employee” button. This will list down the validation messages that last name and email fields can not be submitted blank.
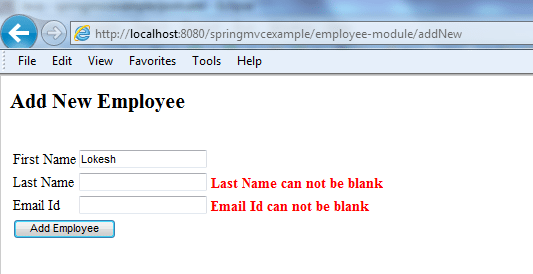
3) Now fill all three values correctly, and submit the form. Now you will be able to see the success message.
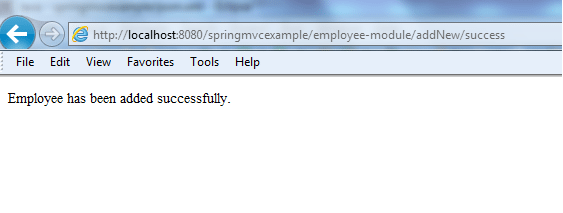
That’s all for this basic but important spring mvc crud example about form submission in spring MVC. Keep me posting about your queries and suggestions.
No comments:
Post a Comment