The Spring MVC form checkbox facilitates to choose multiple options at the same time. This tag renders an HTML input tag of type checkbox.
Syntax
Apart from checkbox tag, Spring MVC form tag library also contains checkboxes tag. This tag renders multiple HTML input tags with type checkbox. This tag is used only if you don't want to list all the elements in the view page. In such a case, you can provide the elements at runtime and pass it to the tag. As the user can select multiple options, you need to pass the elements of Array, a List or a Map type.
Syntax
Example of Spring MVC Form Check Box
1. Add dependencies to pom.xml file.
2. Create the bean class
Reservation.java
3. Create the controller class
ReservationController.java
4. Provide the entry of controller in the web.xml file
web.xml
5. Define the bean in the xml file
spring-servlet.xml
6. Create the requested page
index.jsp
7. Create the view components
reservation-page.jsp
confirmation-page.jsp
Output:
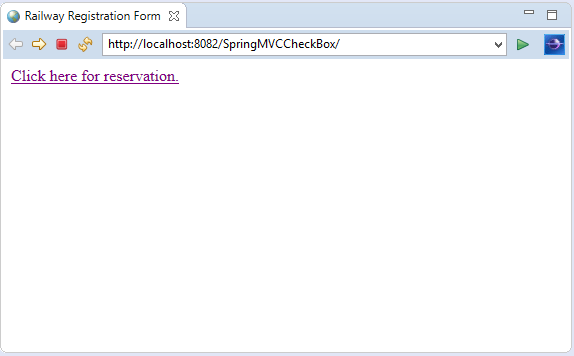



No comments:
Post a Comment