Spring provides integration support with apache tiles framework. So we can simply manage the layout of the Spring MVC application with the help of spring tiles support.
Advantage of Tiles support in Spring MVC
Reusability: We can reuse a single component in multiple pages like header and footer components.
Centralized control: We can control the layout of the page by a single template page only.
Easy to change the layout: By the help of single template page, we can change the layout of the page anytime. So your website can easily adopt new technologies such as bootstrap, jQuery, etc.
Directory Structure
Let's see the files of spring tiles example in eclipse.

Spring MVC Tiles Example
1. Add dependencies to pom.xml file.
pom.xml
- <!-- https://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
- <dependency>
- <groupId>org.springframework</groupId>
- <artifactId>spring-webmvc</artifactId>
- <version>5.1.1.RELEASE</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/javax.servlet/javax.servlet-api -->
- <dependency>
- <groupId>javax.servlet</groupId>
- <artifactId>servlet-api</artifactId>
- <version>3.0-alpha-1</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/javax.servlet/jstl -->
- <dependency>
- <groupId>javax.servlet</groupId>
- <artifactId>jstl</artifactId>
- <version>1.2</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/org.apache.tomcat/tomcat-jasper -->
- <dependency>
- <groupId>org.apache.tomcat</groupId>
- <artifactId>tomcat-jasper</artifactId>
- <version>9.0.12</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/org.apache.tiles/tiles-jsp -->
- <dependency>
- <groupId>org.apache.tiles</groupId>
- <artifactId>tiles-jsp</artifactId>
- <version>3.0.5</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/org.apache.tiles/tiles-servlet -->
- <dependency>
- <groupId>org.apache.tiles</groupId>
- <artifactId>tiles-servlet</artifactId>
- <version>3.0.5</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/org.apache.tiles/tiles-core -->
- <dependency>
- <groupId>org.apache.tiles</groupId>
- <artifactId>tiles-core</artifactId>
- <version>3.0.5</version>
- </dependency>
- <!-- https://mvnrepository.com/artifact/org.apache.tiles/tiles-el -->
- <dependency>
- <groupId>org.apache.tiles</groupId>
- <artifactId>tiles-el</artifactId>
- <version>3.0.5</version>
- </dependency>
2. Create the bean class
Contact.java
- package com.javatpoint.form;
- public class Contact {
- private String firstname;
- private String lastname;
- private String email;
- private String telephone;
- public String getEmail() {
- return email;
- }
- public String getTelephone() {
- return telephone;
- }
- public void setEmail(String email) {
- this.email = email;
- }
- public void setTelephone(String telephone) {
- this.telephone = telephone;
- }
- public String getFirstname() {
- return firstname;
- }
- public String getLastname() {
- return lastname;
- }
- public void setFirstname(String firstname) {
- this.firstname = firstname;
- }
- public void setLastname(String lastname) {
- this.lastname = lastname;
- }
- }
3. Create the controller class
HelloWorldController.java
- package com.javatpoint.controller;
- import org.springframework.stereotype.Controller;
- import org.springframework.ui.Model;
- import org.springframework.web.bind.annotation.RequestMapping;
- @Controller
- public class HelloWorldController {
- @RequestMapping("/hello")
- public String helloWorld(Model m) {
- String message = "Hello World, Spring MVC @ Javatpoint";
- m.addAttribute("message", message);
- return "hello";
- }
- }
ContactController.java
- package com.javatpoint.controller;
- import org.springframework.stereotype.Controller;
- import org.springframework.ui.Model;
- import org.springframework.validation.BindingResult;
- import org.springframework.web.bind.annotation.ModelAttribute;
- import org.springframework.web.bind.annotation.RequestMapping;
- import org.springframework.web.bind.annotation.RequestMethod;
- import org.springframework.web.bind.annotation.SessionAttributes;
- import com.javatpoint.form.Contact;
- @Controller
- @SessionAttributes
- public class ContactController {
- @RequestMapping(value = "/addContact", method = RequestMethod.POST)
- public String addContact(@ModelAttribute("contact") Contact contact, BindingResult result) {
- //write the code here to add contact
- return "redirect:contact.html";
- }
- @RequestMapping("/contact")
- public String showContacts(Model m) {
- m.addAttribute("command", new Contact());
- return "contact";
- }
- }
4. Provide the entry of controller in the web.xml file
web.xml
- <?xml version="1.0" encoding="UTF-8"?>
- <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5">
- <display-name>SpringTiles</display-name>
- <welcome-file-list>
- <welcome-file>index.jsp</welcome-file>
- </welcome-file-list>
- <servlet>
- <servlet-name>spring</servlet-name>
- <servlet-class>
- org.springframework.web.servlet.DispatcherServlet
- </servlet-class>
- <load-on-startup>1</load-on-startup>
- </servlet>
- <servlet-mapping>
- <servlet-name>spring</servlet-name>
- <url-pattern>*.html</url-pattern>
- </servlet-mapping>
- </web-app>
5. Define the bean in the xml file
spring-servlet.xml
- <beans xmlns="http://www.springframework.org/schema/beans"
- xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
- xmlns:p="http://www.springframework.org/schema/p"
- xmlns:context="http://www.springframework.org/schema/context"
- xsi:schemaLocation="http://www.springframework.org/schema/beans
- http://www.springframework.org/schema/beans/spring-beans-3.0.xsd
- http://www.springframework.org/schema/context
- http://www.springframework.org/schema/context/spring-context-3.0.xsd">
- <context:annotation-config />
- <context:component-scan base-package="com.javatpoint.controller" />
- <bean id="viewResolver" class="org.springframework.web.servlet.view.tiles3.TilesViewResolver"/>
- <bean id="tilesConfigurer" class="org.springframework.web.servlet.view.tiles3.TilesConfigurer">
- <property name="definitions">
- <list>
- <value>/WEB-INF/tiles.xml</value>
- </list>
- </property>
- </bean>
- </beans>
6. Provide the tiles.xml file
tiles.xml
- <?xml version="1.0" encoding="UTF-8" ?>
- <!DOCTYPE tiles-definitions PUBLIC
- "-//Apache Software Foundation//DTD Tiles Configuration 2.0//EN"
- "http://tiles.apache.org/dtds/tiles-config_2_0.dtd">
- <tiles-definitions>
- <definition name="base.definition"
- template="/WEB-INF/jsp/layout.jsp">
- <put-attribute name="title" value="" />
- <put-attribute name="header" value="/WEB-INF/jsp/header.jsp" />
- <put-attribute name="menu" value="/WEB-INF/jsp/menu.jsp" />
- <put-attribute name="body" value="" />
- <put-attribute name="footer" value="/WEB-INF/jsp/footer.jsp" />
- </definition>
- <definition name="contact" extends="base.definition">
- <put-attribute name="title" value="Contact Manager" />
- <put-attribute name="body" value="/WEB-INF/jsp/contact.jsp" />
- </definition>
- <definition name="hello" extends="base.definition">
- <put-attribute name="title" value="Hello Spring MVC" />
- <put-attribute name="body" value="/WEB-INF/jsp/hello.jsp" />
- </definition>
- </tiles-definitions>
7. Create the requested page
index.jsp
8. Create the other view components
hello.jsp
contact.jsp
- <%@taglib uri="http://www.springframework.org/tags/form" prefix="form"%>
- <html>
- <head>
- <title>Spring Tiles Contact Form</title>
- </head>
- <body>
- <h2>Contact Manager</h2>
- <form:form method="post" action="addContact.html">
- <table>
- <tr>
- <td><form:label path="firstname">First Name</form:label></td>
- <td><form:input path="firstname" /></td>
- </tr>
- <tr>
- <td><form:label path="lastname">Last Name</form:label></td>
- <td><form:input path="lastname" /></td>
- </tr>
- <tr>
- <td><form:label path="lastname">Email</form:label></td>
- <td><form:input path="email" /></td>
- </tr>
- <tr>
- <td><form:label path="lastname">Telephone</form:label></td>
- <td><form:input path="telephone" /></td>
- </tr>
- <tr>
- <td colspan="2">
- <input type="submit" value="Add Contact"/>
- </td>
- </tr>
- </table>
- </form:form>
- </body>
- </html>
header.jsp
footer.jsp
menu.jsp
layout.jsp
- <%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles"%>
- <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN"
- "http://www.w3.org/TR/html4/loose.dtd">
- <html>
- <head>
- <meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
- <title><tiles:insertAttribute name="title" ignore="true" /></title>
- </head>
- <body>
- <div><tiles:insertAttribute name="header" /></div>
- <div style="float:left;padding:10px;width:15%;"><tiles:insertAttribute name="menu" /></div>
- <div style="float:left;padding:10px;width:80%;border-left:1px solid pink;">
- <tiles:insertAttribute name="body" /></div>
- <div style="clear:both"><tiles:insertAttribute name="footer" /></div>
- </body>
- </html>
Output:
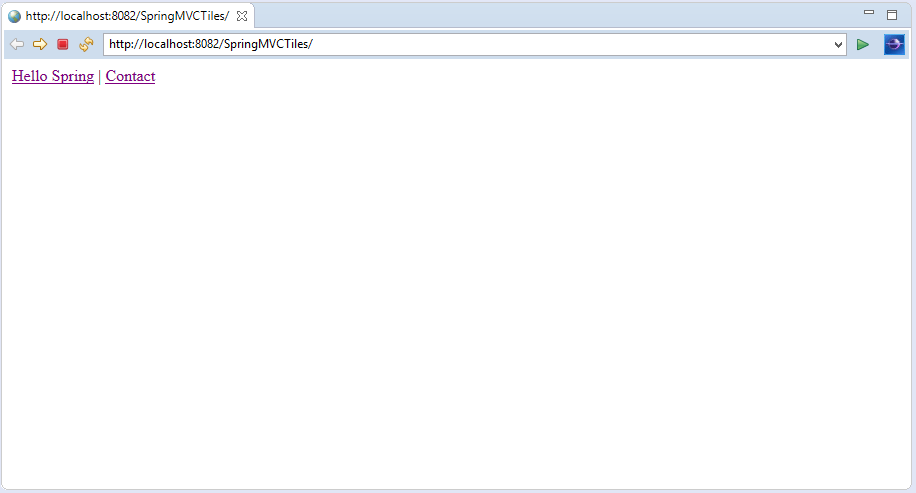

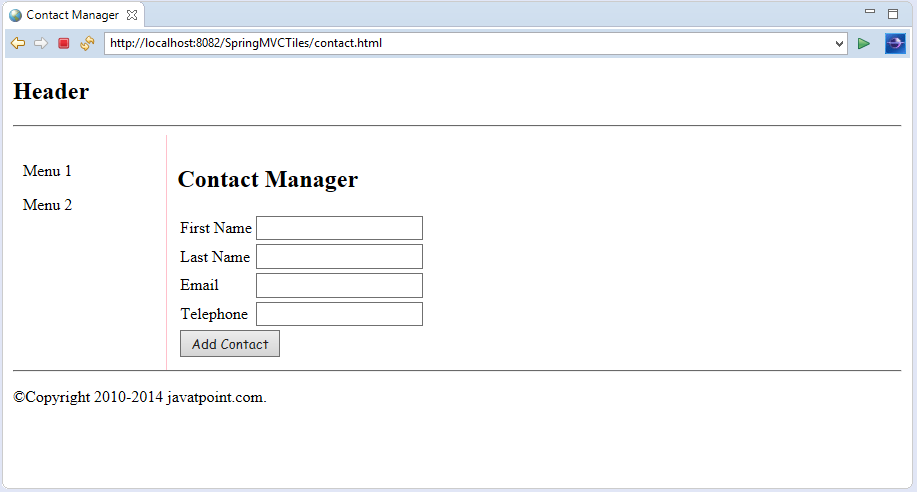
No comments:
Post a Comment