In android, we can easily make a phone call from our android applications by invoking built-in phone calls app using Intents action (ACTION_CALL).
Generally, the Intent object in android with proper action (ACTION_CALL) and data will help us to launch a built-in phone calls app to make a phone calls in our application.
In android, Intent is a messaging object which is used to request an action from another app component such as activities, services, broadcast receivers and content providers. To know more about Intent object in android check this Android Intents with Examples.
To make a phone call using Intent object in android application, we need to write the code like as shown below.
Intent callIntent = new Intent(Intent.ACTION_CALL);
callIntent.setData(Uri.parse("tel:" + txtPhone.getText().toString()));
startActivity(callIntent);
callIntent.setData(Uri.parse("tel:" + txtPhone.getText().toString()));
startActivity(callIntent);
If you observe above code, we are using Intent object ACTION_CALL action to make a phone call based on our requirements.
Now we will see how to make a phone call in android application using Intent action (ACTION_CALL) with examples.
Android Phone Call Example
Following is the example of making a phone call by invoking default phone calls app using Intent object in android application.
Create a new android application using android studio and give names as PhoneCallExample. In case if you are not aware of creating an app in android studio check this article Android Hello World App.
activity_main.xml
<?xml version="1.0" encoding="utf-8"?><LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/fstTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:layout_marginTop="150dp"
android:text="Mobile No"
/>
<EditText
android:id="@+id/mblTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:ems="10">
</EditText>
<Button
android:id="@+id/btnCall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:text="Call" />
</LinearLayout>
android:orientation="vertical" android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:id="@+id/fstTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:layout_marginTop="150dp"
android:text="Mobile No"
/>
<EditText
android:id="@+id/mblTxt"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:ems="10">
</EditText>
<Button
android:id="@+id/btnCall"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginLeft="100dp"
android:text="Call" />
</LinearLayout>
Now open our main activity file MainActivity.java from \src\main\java\com.tutlane.phonecallexample path and write the code like as shown below
MainActivity.java
package com.tutlane.phonecallexample;
import android.Manifest;import android.content.Intent;import android.content.pm.PackageManager;import android.net.Uri;import android.os.Build;import android.support.v4.app.ActivityCompat;import android.support.v7.app.AppCompatActivity;import android.os.Bundle;import android.view.View;import android.widget.Button;import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
private EditText txtPhone;
private Button btn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
txtPhone = (EditText)findViewById(R.id.mblTxt);
btn = (Button)findViewById(R.id.btnCall);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
callPhoneNumber();
}
});
}
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults)
{
if(requestCode == 101)
{
if(grantResults[0] == PackageManager.PERMISSION_GRANTED)
{
callPhoneNumber();
}
}
}
public void callPhoneNumber()
{
try
{
if(Build.VERSION.SDK_INT > 22)
{
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.CALL_PHONE) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.CALL_PHONE}, 101);
return;
}
Intent callIntent = new Intent(Intent.ACTION_CALL);
callIntent.setData(Uri.parse("tel:" + txtPhone.getText().toString()));
startActivity(callIntent);
}
else {
Intent callIntent = new Intent(Intent.ACTION_CALL);
callIntent.setData(Uri.parse("tel:" + txtPhone.getText().toString()));
startActivity(callIntent);
}
}
catch (Exception ex)
{
ex.printStackTrace();
}
}
}
import android.Manifest;import android.content.Intent;import android.content.pm.PackageManager;import android.net.Uri;import android.os.Build;import android.support.v4.app.ActivityCompat;import android.support.v7.app.AppCompatActivity;import android.os.Bundle;import android.view.View;import android.widget.Button;import android.widget.EditText;
public class MainActivity extends AppCompatActivity {
private EditText txtPhone;
private Button btn;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
txtPhone = (EditText)findViewById(R.id.mblTxt);
btn = (Button)findViewById(R.id.btnCall);
btn.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
callPhoneNumber();
}
});
}
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults)
{
if(requestCode == 101)
{
if(grantResults[0] == PackageManager.PERMISSION_GRANTED)
{
callPhoneNumber();
}
}
}
public void callPhoneNumber()
{
try
{
if(Build.VERSION.SDK_INT > 22)
{
if (ActivityCompat.checkSelfPermission(this, Manifest.permission.CALL_PHONE) != PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(MainActivity.this, new String[]{Manifest.permission.CALL_PHONE}, 101);
return;
}
Intent callIntent = new Intent(Intent.ACTION_CALL);
callIntent.setData(Uri.parse("tel:" + txtPhone.getText().toString()));
startActivity(callIntent);
}
else {
Intent callIntent = new Intent(Intent.ACTION_CALL);
callIntent.setData(Uri.parse("tel:" + txtPhone.getText().toString()));
startActivity(callIntent);
}
}
catch (Exception ex)
{
ex.printStackTrace();
}
}
}
If you observe above code we are adding Runtime permissions to make sure our application work in both old / latest android OS versions and we used Intent action (ACTION_CALL) to make phone call on button click using default phone calls app. As discussed, we need to add a CALL_PHONE permission in our android manifest.
Now open android manifest file (AndroidManifest.xml) and write the code like as shown below
AndroidManifest.xml
<?xml version="1.0" encoding="utf-8"?><manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.tutlane.phonecallexample">
<uses-permission android:name="android.permission.CALL_PHONE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
package="com.tutlane.phonecallexample">
<uses-permission android:name="android.permission.CALL_PHONE" />
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/AppTheme">
<activity android:name=".MainActivity">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
If you observe above AndroidManifest.xml file we added a CALL_PHONE permission in manifest file.
Output of Android Phone Call Example
When we run above program in android studio we will get the result like as shown below.
Once we enter phone number and click on Call button, it will invoke built-in phone calls app and it will make a call to respective phone number like as shown below.
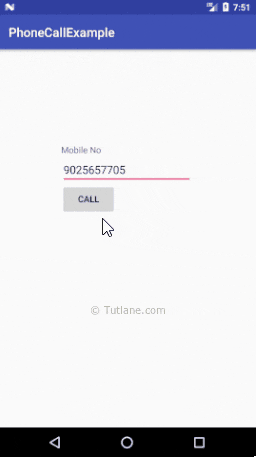
This is how we can make a phone calls using Intents in android applications based on our requirements.
No comments:
Post a Comment